How to Adjust Background Image Opacity in CSS
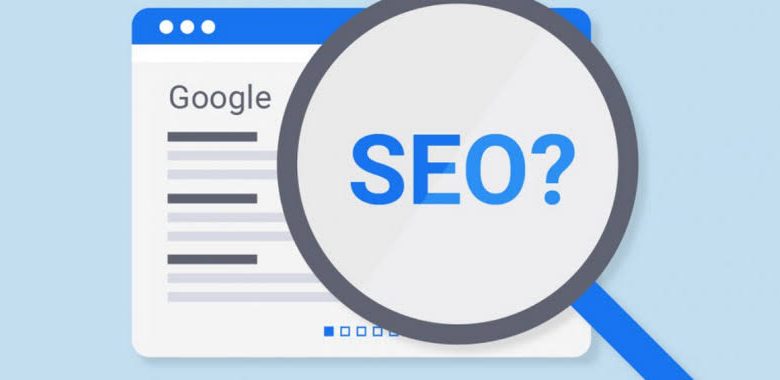
Introduction
opacity is a CSS property that allows you to change the opaqueness of an element. By default, all elements have a value of 1. By changing this value closer to 0, the element will appear more and more transparent.
A common use case is using an image as part of the background. Adjusting the opacity can improve the legibility of text or achieve the desired appearance. However, there is no way to target the background-image of an element with opacity without affecting the child elements.
This limitation can be particularly challenging when designing web pages that require a specific visual hierarchy or readability. For instance, you might want a background image to be less prominent so that text or other content on top of it stands out more clearly. Simply applying the opacity property to the entire element will not suffice, as it will also make the text and other child elements transparent.
In this article, you will learn two methods to work around this limitation for background images with opacity. These methods will help you achieve the desired transparency effect on background images without compromising the visibility of the content within the element.
If you would like to follow along with this article, you will need:
The first approach will rely upon two elements. One is a “wrap” that provides a point of reference with position: relative. The second is an img element that appears behind the content with position: absolute and stacking context.
Here is an example of the markup for this approach:
Hello World!
And here are the accompanying styles:
.demo-wrap { overflow: hidden; position: relative; } .demo-bg { opacity: 0.6; position: absolute; left: 0; top: 0; width: 100%; height: auto; } .demo-content { position: relative; }
This markup and styles will produce a result with text on top of an image:
.css-bg-example-1 .demo-wrap { overflow: hidden; position: relative; } .css-bg-example-1 .demo-bg { opacity: 0.6; position: absolute; left: 0; top: 0; width: 100%; height: auto; } .css-bg-example-1 .demo-content { position: relative; } .css-bg-example-1 .demo-content h1 { padding-top: 100px; padding-bottom: 100px; padding-left: 1em; padding-right: 1em; }
Hello World!
The parent demo-wrap
There are some limitations to this approach. It assumes that your image is large enough to accomodate the size of any element. You may need to enforce size limitations to prevent an image from appearing cut off or not covering the entire height of an element. It will also require additional adjustments if you want to control the “background position” and no clean “background repeat” alternative.
The second approach will rely upon pseudo-elements. The :before and :after pseudo-elements are available to most elements. Typically, you would provide a content value and use it to append extra text at the beginning or end. However, it is also possible to provide an empty string and then you can utilize the pseudo-elements for designs.
Here is an example of the markup for this approach:
Hello World!
And here are the accompanying styles:
.demo-wrap { position: relative; } .demo-wrap:before { content: ‘ ‘; display: block; position: absolute; left: 0; top: 0; width: 100%; height: 100%; opacity: 0.6; background-image: url(‘https://assets.digitalocean.com/labs/images/community_bg.png’); background-repeat: no-repeat; background-position: 50% 0; background-size: cover; } .demo-content { position: relative; }
This markup and styles will produce a result with text on top of an image:
.css-bg-example-2 .demo-wrap { position: relative; } .css-bg-example-2 .demo-wrap:before { content: ‘ ‘; display: block; position: absolute; left: 0; top: 0; width: 100%; height: 100%; opacity: 0.6; background-image: url(‘https://assets.digitalocean.com/labs/images/community_bg.png’); background-repeat: no-repeat; background-position: 50% 0; background-size: cover; } .css-bg-example-2 .demo-content { position: relative; } .css-bg-example-2 .demo-content h1 { padding-top: 100px; padding-bottom: 100px; padding-left: 1em; padding-right: 1em; }
Hello World!
The parent demo-wrap
This approach has the advantage of support for other background properties like background-position, background-repeat, and background-size. This approach has the disadvantage of using one of the pseudo-elements which may conflict with another design effect – like a clearfix solution.
When designing web pages, it’s essential to ensure that text remains readable over background images. Overlays can be used to improve readability by adding a semi-transparent layer between the background image and the text. This technique is particularly useful for hero sections, banners, and other areas where text needs to stand out against a visually appealing background.
.example { position: relative; } .example::before { content: ”; position: absolute; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.5); z-index: -1; }
Hover effects can significantly enhance the user experience by providing visual cues and feedback. One common hover effect is changing the opacity of UI elements, such as buttons or icons, to indicate interactivity. By dynamically adjusting opacity on hover, designers can create a sense of depth and visual interest, making the user interface more engaging and responsive.
.example-button { opacity: 0.8; } .example-button:hover { opacity: 1; }
Hero sections and banners are critical components of modern web design, often serving as the first point of contact between the user and the website. Applying transparent backgrounds to these elements can create a sense of continuity and visual flow, allowing the background image to seamlessly integrate with the rest of the layout. This design approach can be particularly effective in creating a modern, sleek look that draws the user’s attention to the content.
.hero-section { position: relative; background-color: rgba(255, 255, 255, 0.5); background-image: url(‘hero-image.jpg’); background-blend-mode: multiply; }
How do I make a background image transparent in CSS?
To make a background image transparent in CSS, you can use the background-blend-mode property in combination with a semi-transparent color. Here’s an example:
div { background-color: rgba(255, 255, 255, 0.5); background-image: url(‘image.jpg’); background-blend-mode: multiply; }
This will make the background image slightly transparent, allowing the background color to show through.
Can I change background image opacity without affecting text?
Yes, you can change the background image opacity without affecting the text by using a pseudo-element or a separate element for the background image. Here’s an example using a pseudo-element:
div { position: relative; } div::before { content: ”; position: absolute; top: 0; left: 0; width: 100%; height: 100%; background-image: url(‘image.jpg’); background-size: cover; opacity: 0.5; z-index: -1; }
This way, the opacity of the background image is adjusted without affecting the text or other content within the element.
What’s the difference between opacity and background-blend-mode?
opacity affects the entire element, including its content, whereas background-blend-mode only affects the background image or color. opacity sets the level of transparency for the entire element, making it and its content semi-transparent. On the other hand, background-blend-mode blends the background image or color with the background color, allowing for more control over the background’s transparency and appearance.
How to make only background opacity in CSS?
To make only the background opaque in CSS, you can use the background-color property with an rgba value, setting the alpha channel to 1 (fully opaque). Here’s an example:
div { background-color: rgba(255, 255, 255, 1); }
This sets the background color to be fully opaque, ensuring that the background is not transparent.
How to make a background image transparent?
To make a background image transparent, you can use the background-blend-mode property in combination with a semi-transparent color, as shown in the first FAQ. Alternatively, you can use a pseudo-element or a separate element for the background image and adjust its opacity property, as shown in the second FAQ.
How do I dull the background image in CSS?
To dull the background image in CSS, you can use the filter property to apply a blur effect. Here’s an example:
div { background-image: url(‘image.jpg’); background-size: cover; filter: blur(8px); }
This adds a blur effect to the background image, making it appear duller. You can adjust the value of blur to control the level of dullness.
In this article, you learned about two methods to work around this limitation for background images with opacity.
For more in-depth information on CSS, you can use the following tutorials:
Additionally, if you’d like to deep-dive into CSS, check out our learn more about CSS series for exercises and programming projects.