How to Check if Two Strings Are Equal in Python (With Examples)
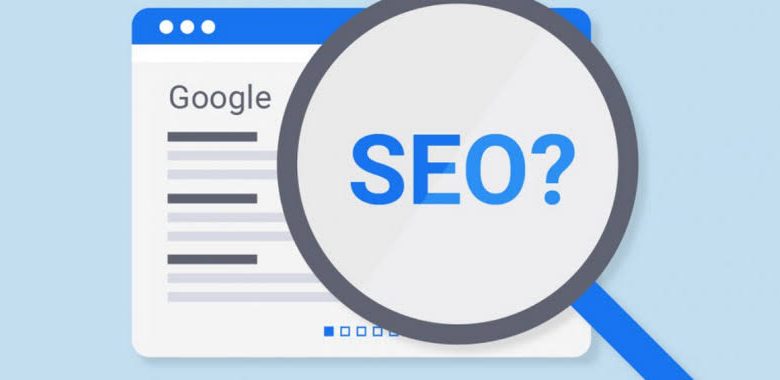
Introduction
Python strings equality can be checked using == operator or __eq__() function. Python strings are case sensitive, so these equality check methods are also case sensitive.
Let’s look at some examples to check if two strings are equal or not.
s1 = ‘Apple’ s2 = ‘Apple’ s3 = ‘apple’ if s1 == s2: print(‘s1 and s2 are equal.’) if s1.__eq__(s2): print(‘s1 and s2 are equal.’)
Output:
s1 and s2 are equal. s1 and s2 are equal.
If you want to perform inequality check, you can use != operator.
if s1 != s3: print(‘s1 and s3 are not equal’)
Output: s1 and s3 are not equal
Sometimes we don’t care about the case while checking if two strings are equal, we can use casefold(), lower() or upper() functions for case-insensitive equality check.
if s1.casefold() == s3.casefold(): print(s1.casefold()) print(s3.casefold()) print(‘s1 and s3 are equal in case-insensitive comparison’) if s1.lower() == s3.lower(): print(s1.lower()) print(s3.lower()) print(‘s1 and s3 are equal in case-insensitive comparison’) if s1.upper() == s3.upper(): print(s1.upper()) print(s3.upper()) print(‘s1 and s3 are equal in case-insensitive comparison’)
Output:
apple apple s1 and s3 are equal in case-insensitive comparison apple apple s1 and s3 are equal in case-insensitive comparison APPLE APPLE s1 and s3 are equal in case-insensitive comparison
Let’s look at some examples where strings contain special characters.
s1 = ‘$#ç∂’ s2 = ‘$#ç∂’ print(‘s1 == s2?’, s1 == s2) print(‘s1 != s2?’, s1 != s2) print(‘s1.lower() == s2.lower()?’, s1.lower() == s2.lower()) print(‘s1.upper() == s2.upper()?’, s1.upper() == s2.upper()) print(‘s1.casefold() == s2.casefold()?’, s1.casefold() == s2.casefold())
Output:
s1 == s2? True s1 != s2? False s1.lower() == s2.lower()? True s1.upper() == s2.upper()? True s1.casefold() == s2.casefold()? True
That’s all for checking if two strings are equal or not in Python.
You can checkout complete script and more Python String examples from our GitHub Repository.
The == operator checks for exact equality, including case sensitivity. The .lower() and .casefold() methods, on the other hand, perform case-insensitive comparisons. The difference between the two is that .lower() is not suitable for all Unicode characters, while .casefold() is.
Example:
s1 = ‘Apple’ s2 = ‘apple’ print(s1 == s2) print(s1.lower() == s2.lower()) print(s1.casefold() == s2.casefold())
The is operator checks for object identity, not equality. It’s important to avoid using is for string comparison, as it can lead to unexpected results. Instead, use the == operator for string comparison.
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 is s2) print(s1 == s2)
When comparing strings with special characters or Unicode, it’s important to use the appropriate method for your use case. If you need a case-insensitive comparison, use .casefold(). If you need a case-sensitive comparison, use ==.
Example:
s1 = ‘ççç’ s2 = ‘ÇÇÇ’ print(s1 == s2) print(s1.casefold() == s2.casefold())
Using is instead of == for string comparison
This is a common mistake when comparing strings in Python. The is operator checks for object identity, not equality. To fix this, use the == operator for string comparison.
Example of the mistake:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 is s2)
Corrected code:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2)
Comparing string to None or non-string types
When comparing a string to None or non-string types, Python will raise a TypeError. To avoid this, make sure to compare strings to other strings or to None explicitly.
Example of the mistake:
s1 = ‘Hello’ s2 = None print(s1 == s2)
Corrected code:
s1 = ‘Hello’ s2 = None if isinstance(s2, str): print(s1 == s2) else: print(“s2 is not a string.”)
Misleading results due to trailing/leading whitespace
Trailing or leading whitespace can lead to misleading results when comparing strings. To fix this, use the strip() method to remove any leading or trailing whitespace before comparing strings.
Example of the mistake:
s1 = ‘Hello’ s2 = ‘ Hello ‘ print(s1 == s2)
Corrected code:
s1 = ‘Hello’ s2 = ‘ Hello ‘ print(s1 == s2.strip())
1. Can you use == for strings in Python?
Yes, you can use the == operator to compare strings in Python. This operator checks if the values of the strings are equal.
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2)
2. How do you check if a string is equal in Python?
You can check if a string is equal in Python by using the == operator. This operator checks if the values of the strings are equal.
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2)
3. How do you check if a variable is equal to a string in Python?
You can check if a variable is equal to a string in Python by using the == operator. This operator checks if the value of the variable is equal to the string.
Example:
var = ‘Hello’ print(var == ‘Hello’)
4. Do you use == for strings?
Yes, you use the == operator for strings in Python. This operator checks if the values of the strings are equal.
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2)
5. How do I check if two strings are equal in Python?
You can check if two strings are equal in Python by using the == operator. This operator checks if the values of the strings are equal.
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2)
6. What’s the difference between == and is in Python?
The == operator checks if the values of two objects are equal, while the is operator checks if two objects are the same (i.e., they refer to the same memory location).
Example:
s1 = ‘Hello’ s2 = ‘Hello’ print(s1 == s2) print(s1 is s2)
7. How do I do a case-insensitive string comparison in Python?
You can do a case-insensitive string comparison in Python by converting both strings to lowercase or uppercase using the lower() or upper() method, respectively.
Example:
s1 = ‘Hello’ s2 = ‘hello’ print(s1.lower() == s2.lower())
8. Why is my string comparison not working?
Your string comparison might not be working due to various reasons such as:
- Case sensitivity: Strings are case sensitive, so ‘Hello’ and ‘hello’ are not equal.
- Leading or trailing whitespace: Strings with leading or trailing whitespace are not equal to strings without them.
- Non-string comparison: Comparing a string to a non-string object will raise a TypeError.
Example:
s1 = ‘Hello’ s2 = ‘hello’ print(s1 == s2)
9. Can I compare a string to None in Python?
Yes, you can compare a string to None in Python. However, you should ensure that the object you are comparing is a string or None explicitly to avoid TypeError.
Example:
s1 = ‘Hello’ s2 = None if isinstance(s2, str): print(s1 == s2) else: print(“s2 is not a string.”)
10. How to compare multi-line strings in Python?
You can compare multi-line strings in Python by using the == operator. However, ensure that the strings are formatted correctly, including any newline characters.
Example:
s1 = “””Hello World””” s2 = “””Hello World””” print(s1 == s2)
In this comprehensive guide, we have delved into the intricacies of string comparison in Python. We began by exploring the fundamental methods for checking string equality, including the use of the == operator and the __eq__() function. We also highlighted the importance of considering case sensitivity in string comparisons and demonstrated how to perform case-insensitive comparisons using the lower(), upper(), and casefold() methods.
Furthermore, we discussed the key differences between the == operator and the is operator, emphasizing the importance of using == for string comparison to ensure accurate results. Additionally, we touched upon common errors that can occur during string comparison, such as ignoring case sensitivity or comparing strings to non-string objects, and provided valuable debugging tips to overcome these issues.
To further enhance your understanding of string manipulation in Python, we recommend exploring the following tutorials:
By reading these tutorials, you’ll gain a deeper understanding of string manipulation and comparison in Python, enabling you to write more efficient and effective code.